Last updated: 09/03/2024
Traditionally menus, used for navigating a website, are designed using CSS. Pure CSS menus are simple, looks good and it helps laying out the elements properly on a website. Here, in this article I am going to show you, with an example, on how to design a cool vertical accordion menu using jQuery and CSS.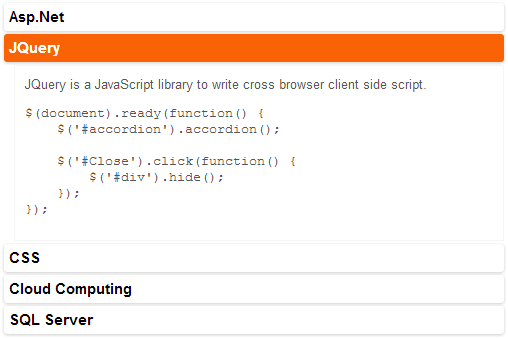
An accordion menu is useful when there is very little space on a web page. You can hide paragraphs and important contents inside the accordion. Using jQuery .accordion() function, you can easily add a simple accordion menu on your web page. Further, using CSS you can style it to give it nice finish.
To design the menu, I’ll first add a <div> and <h3> element on the web page. The <div> works as the container and it will hold the contents. The <h3> (header) element will serve as our menu heads.
<body> <div id="accordion"> <h3>Asp.Net</h3> <div>Hello World (C#) <pre> using System; protected void Page_Load(object sender, EventArgs e) { Response.Write("Hello, world"); } </pre> </div> <h3>jQuery</h3> <div>jQuery is a JavaScript library to write cross browser client side script. <pre> $(document).ready(function() { $('#accordion').accordion(); $('#Close').click(function() { $('#div').hide(); }); }); </pre> </div> <h3>CSS</h3> <div>Cascading Style Sheets (CSS) is used for styling and laying HTML elements on a web page. <pre> .classname div { padding:3px 5px; border:solid 1px #CCC } </pre> </div> <h3>Ajax</h3> <div>Ajax (Asynchronous JavaScript and XML) allows Web applications to send and receive data from a server, asynchronously. It is a clean way of communicating with a server, without refreshing the existing page. </div> <h3>SQL Server</h3> <div>Microsoft's SQL Server is a Relational Database Managment System (RDBMS), whose primary function is to store and retrieve various types of data. <pre> SELECT EmpID, EmpName, Designation FROM Employee WHERE EmpID = 3</pre></div> </div> </body>
Add Style to the menu
I have kept the CSS as simple as possible, since we will focus more on the jQuery function and the scripts to make the menus expand (or collapse). The style will give it a smooth flow and also highlight (or color) the active header.
<head> <style> :focus, :active { outline:0; } #accordion { width:500px; margin:0 auto; font:normal normal 13px sans-serif; color:#555; } #accordion h3 { padding:5px; -webkit-border-radius:3px; border-radius:3px; -moz-box-shadow:0 1px 4px #CCC; -webkit-box-shadow:0 1px 4px #CCC; box-shadow:0 1px 4px #CCC; margin:3px 0; cursor:pointer; } #accordion div { margin:0 0 0 10px; padding:10px; border:solid 1px #F6F6F6; height:auto; } </style> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script> <script src="https://ajax.googleapis.com/ajax/libs/jqueryui/1.10.2/jquery-ui.min.js"></script> </head>
jQuery .accordion() function
Finally, we will write the script that will make the menus work the way we want it. I'll add animation and color using jQuery .css() method.
The click event of each menu header will result in expanding or collapsing of the menus. In addition, I want to highlight the headers to avoid any confusion about the active menu block.
<script> $(document).ready(function() { // jQuery function. $("#accordion").accordion({ heightStyle: "content" }); }); // EXPAND AND COLLAPSE MENUS ON “HEADER CLICK” $("#accordion h3").click(function() { $("#accordion h3").animate({ 'background-color': '#FFF' }, 300); $("#accordion h3").css({ 'color': '#000' }); // HIGHLIGHT THE SELECTED HEADER (BLOCK) $(this).animate({ 'background-color': '#FA6305' }, 300); $(this).css({ 'color': '#FFF' }); }); </script><
It is so simple.
💡 If you have noticed, I didn't do anything to make the accordion. All I have done is, create a container using a DIV element, gave it an ID and added few contents to it. Finally, in the script section, I have simply attached the DIV element to the jQuery .accordion() function. That's it. Rest everything is taken care of by the function (behind the scene).
I want you to check the demo and get a feel of it.