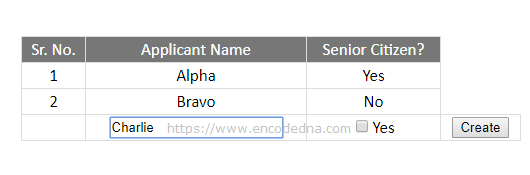
A checkbox is a square box, which you can tick or check based on your requirement. If you have multiple checkboxes on your webpage, you will need to add a label element (next to each square box) to identify the checkboxes.
You can easily add a checkbox with a label at design time. However, adding a checkbox dynamically at runtime, is a slightly tricky process.
Here’s how you should do this.
<html> <head> <style> <!--style HTML table--> table { width: 100%; } table, th, td, th { border: solid 1px #DDD; border-collapse: collapse; padding: 2px 3px; text-align: center; font-weight: normal; } </style> </head> <body> <!--the element to which we'll add the dynamically created table.--> <div id="container" style="width:500px;overflow:auto;"></div> </body> <script> var myApp = new function () { // An array of JSON objects with values. this.arrPack = [{ 'Sr.No.': '', 'Applicant Name': '', 'Senior Citizen?': ''}] this.col = []; this.createTable = function () { for (var i = 0; i < this.arrPack.length; i++) { for (var key in this.arrPack[i]) { if (this.col.indexOf(key) === -1) { this.col.push(key); } } } // Create a table var table = document.createElement('table'); table.setAttribute('id', 'mTable'); var tr = table.insertRow(-1); for (var h = 0; h < this.col.length; h++) { // Add table header. var th = document.createElement('th'); th.innerHTML = this.col[h].replace('_', ' '); tr.appendChild(th); tr.setAttribute('style', 'background-color:#777;color:#fff;'); } // Add new rows to the table using JSON data. for (var i = 1; i < this.arrPack.length; i++) { tr = table.insertRow(-1); for (var j = 0; j < this.col.length; j++) { var tabCell = tr.insertCell(-1); tabCell.innerHTML = this.arrPack[i][this.col[j]]; } } tr = table.insertRow(-1); // Create the last row. // Create and add checkbox and textbox controls to table cells. for (var j = 0; j < this.col.length; j++) { var newCell = tr.insertCell(-1); if (j >= 1) { if (j == 1) { var tBox = document.createElement('input'); tBox.setAttribute('type', 'text'); tBox.setAttribute('value', ''); newCell.appendChild(tBox); } else if (j == 2) { // Here I am creating and adding checkbox to the table cell. var chk = document.createElement('input'); var lbl = document.createElement('label'); chk.setAttribute('type', 'checkbox'); chk.setAttribute('value', 'yes'); chk.setAttribute('id', 'chkIfSenior' + i); lbl.setAttribute('for', 'chkIfSenior' + i); lbl.appendChild(document.createTextNode('Yes')); newCell.appendChild(chk); newCell.appendChild(lbl); } } } this.td = document.createElement('td'); tr.appendChild(this.td); var btNew = document.createElement('input'); btNew.setAttribute('type', 'button'); btNew.setAttribute('value', 'Create'); btNew.setAttribute('id', 'New' + i); btNew.setAttribute('onclick', 'myApp.CreateNew(this)'); this.td.appendChild(btNew); var div = document.getElementById('container'); div.innerHTML = ''; div.appendChild(table); // Add the newlly created table to the page. }; // FUNCTION TO CREATE A NEW TABLE ROW. this.CreateNew = function (oButton) { var activeRow = oButton.parentNode.parentNode.rowIndex; var tab = document.getElementById('mTable').rows[activeRow]; var obj = {}; obj[this.col[0]] = ''; // Add new values to arrPack array. for (i = 1; i < this.col.length; i++) { var td = tab.getElementsByTagName("td")[i]; if (td.childNodes[0].getAttribute('type') == 'text' || td.childNodes[0].getAttribute('type') == 'checkbox') { var txtVal; if (td.childNodes[0].getAttribute('type') == 'checkbox') { // GET THE VALUE OF THE CHECKBOX. if (td.childNodes[0].checked) txtVal = 'Yes'; else txtVal = 'No'; } else { if (td.childNodes[0].value !== '') { txtVal = td.childNodes[0].value; } else { txtVal = ' '; } } if (txtVal != '') { obj[this.col[i]] = txtVal.trim(); } } } obj[this.col[0]] = this.arrPack.length; // The new ID for Sr.No. if (Object.keys(obj).length > 0) { this.arrPack.push(obj); // Add data to the JSON array. this.createTable(); // Refresh the table. } } } myApp.createTable(); </script> </html>
Do not get overwhelmed by the size of the above code. I am creating the table and other elements dynamically and add the table to a div element inside the <body> section.
Let me explain.
I have an array with three columns for the table header. Next, I am creating the table. The code where I am creating and adding the checkbox is this …
else if (j == 2) { var chk = document.createElement('input'); var lbl = document.createElement('label'); chk.setAttribute('type', 'checkbox'); chk.setAttribute('value', 'yes'); chk.setAttribute('id', 'chkIfSenior' + i); lbl.setAttribute('for', 'chkIfSenior' + i); lbl.appendChild(document.createTextNode('Yes')); newCell.appendChild(chk); newCell.appendChild(lbl); }
I am creating two elements in the above code. First, an input element of type checkbox and second a label element for the checkbox name. Both the elements are appended or added to the table cell.
The entire process is repeated when I click the Create button to add a new row to the table.
If you want to learn more about to how to add a dropdown list or a button control to a table, then you must check this post.